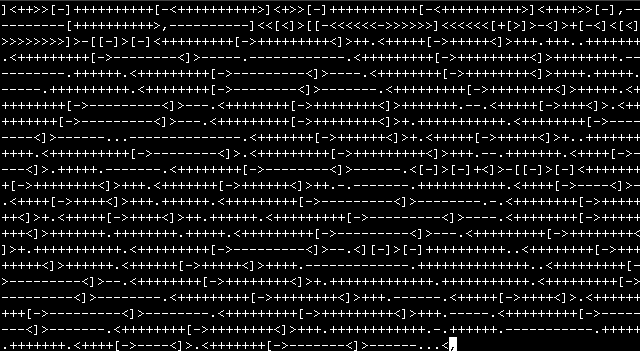
If you earn your bread as a web developer, then you know how frustrating it can be to keep up with all the new libraries and frameworks. If you use jquery and PHP you’re a pleb. HTML? You must be mad. Everyone knows a good developer in 2017 uses Babel, React, ES5, ECMAScript, Browserfy, Webpack and….
Seriously, unless you’re a masochist, there’s no reason why you should dive straight for all the ‘developing trends of 2017’. Stick to core tools and add new ones when you need them and you should be just fine. Coding — writing instructions for a machine — should be made easier, not more confusing. Speaking of which, it seems like Urban Müller missed the memo.
Müller is an underground culture sensation among computer programmers. In 1993, at the time a physics student in Switzerland, the young computer programmer set out to make the smallest possible compiler. The compiler is what translates code written in a programming language into something your CPU can understand, i.e. binary code. The smaller the compiler, the less time and energy it takes for the machine to respond.
In the end, Müller came up with ‘Brainfuck’, a new programming language whose compiler uses only 240 bytes of memory making it around 11,000 times smaller than the C++ compiler. However, by making such a smaller compiler, Müller had to sacrifice practicality. In Brainfuck, you can only use eight commands: <, >, +, –, [, ], and ,. That’s it. Can you imagine writing code just with that? This programming language sticks to its name, that’s for sure, despite being Turing-complete. That was the entire point, in fact — make an esoteric new programming language whose role is not to be of any particular use. It’s simply an amusing challenge.
Let’s take a look at some Brainfuck code. One of the first things a newbie programmer learns to do is display or echo a string of text. Usually, the string ‘Hello, world!’ is used to illustrate basic syntax. To output this text, in Java you have to write:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World"); } }
In Python, it’s this simple:
print “Hello, World!”
In Brainfuck, it’s:
++++++++++[>+++++++>++++++++++>+++>+<<<<-]>++.>+.+++++++..+++.>++.<<+++++++++++++++.>.+++.------.--------.>+.>.
Here’s how you add “5” and “2” together.
++ Cell c0 = 2 > +++++ Cell c1 = 5 [ Start your loops with your cell pointer on the loop counter (c1 in our case) < + Add 1 to c0 > - Subtract 1 from c1 ] End your loops with the cell pointer on the loop counter At this point our program has added 5 to 2 leaving 7 in c0 and 0 in c1 BUT we cannot output this value to the terminal since it's not ASCII encoded! To display the ASCII character "7" we must add 48 to the value 7! 48 = 6 * 8 so let's use another loop to help us! ++++ ++++ c1 = 8 and this will be our loop counter again [ < +++ +++ Add 6 to c0 > - Subtract 1 from c1 ] < . Print out c0 which has the value 55 which translates to "7"!
To understand how to write code in Brainfuck, there’s a great resource over at Stackoverflow and even a Brainfuck for dummies guide.
Though practically impossible to make high-level programs with Brainfuck, theoretically you could design software just as good as anything in C++ or Java. Being Turing complete means the Brainfucked machine can solve any problem given enough time and memory. It’s writing the code that’s challenging.
In the intervening two decades since Müller first designed his programming language, other fellow code alchemists responded with their own versions and derivatives. In DerpPlusPlus some of the commands you can use are ‘HERP’, ‘DERP’, ‘GIGITY’, etc. Ook! maps brainfuck’s eight commands to two-word permutations of “Ook.”, “Ook?”, and “Ook!”, and is supposedly made to be “writable and readable by orang-utans”. For instance, ‘Hello, world!’ in Ook! looks like:
Ook. Ook? Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook! Ook? Ook? Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook? Ook! Ook! Ook? Ook! Ook? Ook. Ook! Ook. Ook. Ook? Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook! Ook? Ook? Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook? Ook! Ook! Ook? Ook! Ook? Ook. Ook. Ook. Ook! Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook! Ook. Ook! Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook! Ook. Ook. Ook? Ook. Ook? Ook. Ook? Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook! Ook? Ook? Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook? Ook! Ook! Ook? Ook! Ook? Ook. Ook! Ook. Ook. Ook? Ook. Ook? Ook. Ook? Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook! Ook? Ook? Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook? Ook! Ook! Ook? Ook! Ook? Ook. Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook. Ook? Ook. Ook? Ook. Ook? Ook. Ook? Ook. Ook! Ook. Ook. Ook. Ook. Ook. Ook. Ook. Ook! Ook. Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook. Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook! Ook. Ook. Ook? Ook. Ook? Ook. Ook. Ook! Ook.
The oddest Brainfuck derivative might be Bodyfuck which uses a gesture-controlled system so that movements are turned into some of the eight available commands. Here’s how you write ‘Hello, world!’ in Bodyfuck.
https://vimeo.com/11976599
There are over 200 esoteric programming languages, many inspired by Brainfuck and some completely hilarious. To print ‘Hello, world!’ in Beatnik you have to write an 80-line poem, in Chef you have to write a recipe, and in DNA# the code is made from strings of A, T, G, C arranged in a helix.